Maven est un outil java de build :
- Gestion de dépendances ;
- Construction multi-module ;
- Orienté plugin et évolutif (des plugin pour modifier le cycle de vie et de compilation, des plugins pour configurer un eclipse).
Plus besoin de créer un script pour construire votre application.
Le fichier POM représente le modèle du projet et décrit :
- Le nom de projet ;
- le type d'artefact ;
- Structure du projet ;
- Dépendances du projet.
Dans le cycle de vie ’par défaut’ d’un projet Maven, les phases les plus utilisées sont :
validate
: vérifie les prérequis d’un projet mavencompile
: compilation du code sourcetest
: lancement des tests unitairespackage
: assemble le code compilé en un livrableinstall
: partage le livrable pour d’autres projets sur le même ordinateurdeploy
: publie le livrable pour d’autres projets dans un repository distant
Les phases s’exécutent de façon séquentielle de façon à ce qu’une phase dépende de la phase précédente.
m2eclipse
édité par Sonatype permet le support de Maven dans l’IDE eclipse. Renommé en m2e
, il est désormais géré par la fondation eclipse.
Notions importantes
Les repositories
Les projets utilisent des librairies. Ces dernières sont stockées dans des espaces qui s’appellent repositories. On distingue 2 types de repositories : les locaux et les distants.
Parmi les repositories distants, il y en a des publics et des privés (repository d’entreprise par exemple qui met à disposition des artifacts au sein de l’entreprise). Les repositories mettent à disposition des librairies (des fichiers JAR mais pas seulement) en téléchargement. On appelle ces libraires artifacts.
Un repository local est une copie en local de repository distant contenant tous les artifacts téléchargés dans le passé (mise en cache).
Un artifact Maven est composé d’un nom de domaine (fr.lps2ima
par exemple), d’un id (contact
par exemple) et d’un numéro de version (0.0.1).
Gestion des dépendances
Sans Maven, vous auriez dû aller dans les propriétés de votre projet dans Eclipse et ajouter vos dépendances dans cet écran :
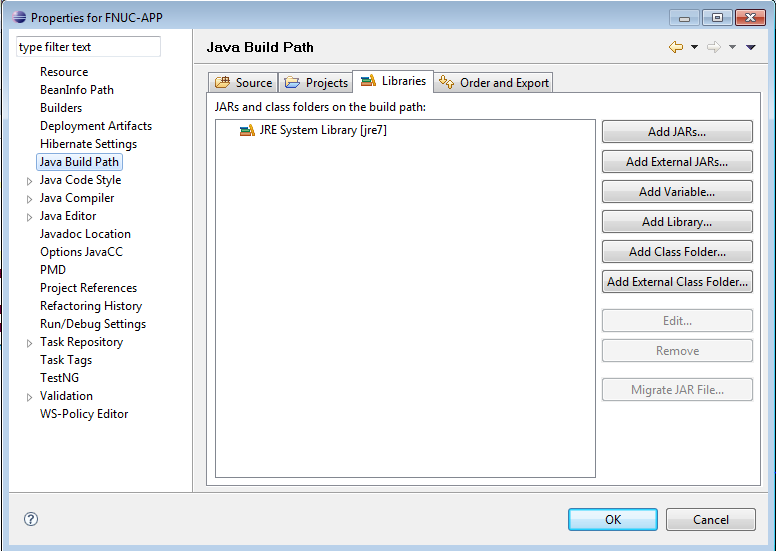
Il faut le faire manuellement pour chaque librairie, et disposer de ces dernières.
Avec Maven, la gestion des dépendances s’effectue au travers d’un fichier bien connu : le pom.xml
. En voici un exemple :
Voici un exemple de fichier pom.xml
:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <name>Contact</name> <artifactId>fr.lps2im.contact</artifactId> <groupId>fr.lps2im</groupId> <version>1.0.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>fr.lps2im.library</groupId> <artifactId>model</artifactId> <version>1.0.0-SNAPSHOT</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.11</version> <scope>test</scope> </dependency> <dependency> <groupId>org.easymock</groupId> <artifactId>easymock</artifactId> <exclusions> <exclusion> <artifactId>cglib</artifactId> <groupId>cglib</groupId> </exclusion> </exclusions> <version>3.3.1</version> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.2</version> <configuration> <source>1.6</source> <target>1.6</target> <encoding>UTF-8</encoding> </configuration> </plugin> </plugins> </build> </project>
Dans l’exemple ci-dessus, la partie au début définit les propriétés de l’artifact (de votre projet du coup). Ce qui est inclus entre <dependencies>
et </dependencies>
sont les dépendances que l’on souhaite importer.
La 1ère dépendance est une dépendance interne (on ne la trouvera pas sur un ''repository public, elle sera sur le repository d’entreprise lps2ima). Les deux autres dépendances par contre sont disponibles sur les repos publics, il s’agit de jUnit et EasyMock.
Dans une invite de commande, un mvn validate
se chargera de vérifier la cohérence du pom
mais surtout (et c’est ce qui nous intéresse ici), ramènera les dépendances sur votre projet. Il ira chercher sur le ou les repositories configurés (on va dire ici qu’il s’agit du repository d’entreprise) les artifacts dans les versions spécifiées, puis ira les copier dans votre repository local. Si vous avez déjà la dernière version d’un artifact en local, il ira directement la chercher là-bas.
Dépendances transitives
Nous avons ajouté une dépendance vers la libraire fr.s2ima.library.model 0.0.1-SNAPSHOT. Cette librairie contient elle aussi un fichier pom.xml
avec ses dépendances. Ces dépendances sont ce qu’on appelle “transitives”, elles sont indirectes.
Sans Maven, à l’exécution de votre projet, vous aurez une erreur disant qu’il manque des dépendances. Il faudra aller les chercher manuellement et vérifier qu’il n’y a pas de conflits de versions. Cela va vite devenir fastidieux.
Avec Maven, ces dépendances seront ramenées automatiquement en cascade.
La commande mvn dependency:tree
vous permet de voir l’arbre des dépendances de votre projet.
Déploiement des artifacts
Pour mettre à disposition votre artifact sur le repository distant configuré, vous devez appeler la commande mvn deploy
.
Repartons sur nos dépendances (voir schéma ci-dessus). Nous travaillons sur la librairie “contact” qui utilise “model”. Or, nous souhaitons ajouter une fonctionnalité dans “model” qui sera utilisée dans “commons”.
Nous pourrions faire l’évolution dans “model” puis lancer un mvn deploy
afin que la dépendance soit accessible dans “contact”. Si vous êtes sûr de votre coup et que vos tests sont suffisant pour déterminer que le travail est bien fait, pourquoi pas. Sinon, il serait plus pratique de pousser dans votre repository local afin de rendre la dépendance visible à vos autres projets, tester, puis mettre à disposition sur le repository distant une fois satisfait.
C’est ce que fait la commande mvn install
. Elle va compiler, exécuter les tests, puis déployer dans le repository local. Vous pourrez donc tester sereinement votre dépendance en local avant de la mettre à disposition avec mvn deploy
.
Ce n’est pas tout
Maven est une boite à outils qui permet de faire beaucoup d’autres choses. Il existe des plugins dont la configuration se fait toujours dans le pom.xml
et qui permet d’automatiser beaucoup de tâches : compilation (mvn compile
), exécution des tests (mvn test
), génération de la javadoc, etc…
Vous pouvez (devez) également factoriser vos dépendances avec l’utilisation de pom parent, faire des modules pour lier vos projets, gérer vos releases, etc…
Pour finir, il existe un plugin dans eclipse (m2) qui vous affranchit de toutes ces commandes. Il me semble cependant nécessaire de bien comprendre le fonctionnement de Maven avant d’utiliser ces outils.
Quelques recommandations concernant le réseau de l'Université de Bretagne-Sud
Eclipse doit avoir la configuration du proxy web pour télécharger des plugins.
Windows
> Preferences
> General
> Network Connections
.
Entrez les valeurs pour les protocoles HTTP et HTTPS.
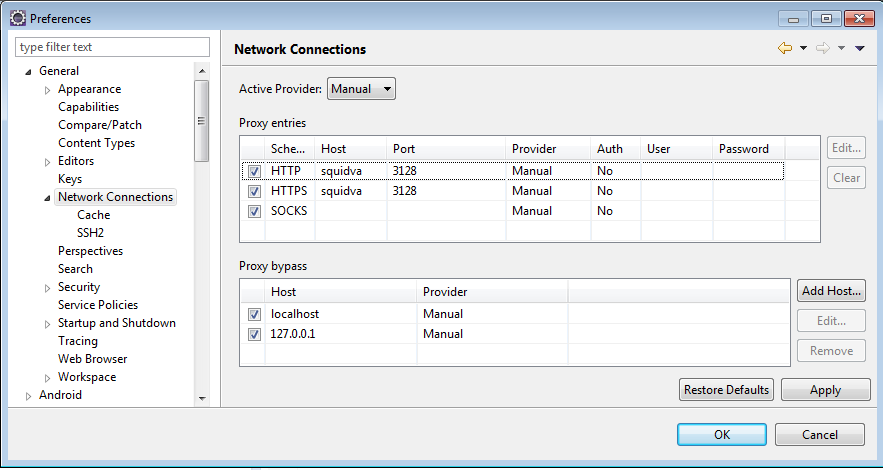
Attention le workspace sur un poste enseignement doit être écrit sur le lecteur D:
ou H:
ou P:
. Si il est dans un sous répertoire de C:\Users\*
, il sera effacé à la fermeture de la session Windows 7.
Sur le réseau ENSEIGNEMENT de l'Université de Bretagne-Sud, le proxy/cache doit être signalé à Maven par l'intermédiaire du fichier
. Il faut l'éditer. Ce fichier n'est pas à modifier lorsque l'on est sur le réseau wifi eduroam.
%USERPROFILE%\.m2\settings.xml
<settings> <proxies> <proxy> <active>true</active> <protocol>http</protocol> <host>squidva</host> <port>3128</port> </proxy> </proxies> </settings>
Vous pouvez télécharger le fichier de configuration du proxy du réseau ENSEIGNEMENT de l'université de Bretagne-Sud pour Maven à placer dans %USERPROFILE%\.m2
Fichier de configuration du proxy du réseau ENSEIGNEMENT de l'université de Bretagne-Sud pour Maven ()
plugin Eclipse
m2eclipse
édité par Sonatype permet le support de Maven dans l’IDE eclipse. Renommé en m2e
, il est désormais géré par la fondation eclipse.
Ce plugin apporte des fonctionnalités notamment au niveau de l’édition des fichiers pom.xml
, de la gestion et de l’actualisation des dépendances du projet dans Eclipse et de la gestion du filtrage des fichiers de ressources.
Ce plugin rajoute plusieurs menus :
- Pour créer de nouveau projet avec des assistants en faisant [
File
>New
>Other...
>Maven
]. - Pour importer des projet Maven déjà existant en faisant [
File
>Import...
>Maven
]. - Pour configurer Maven en faisant [
Window
>Preference
>Maven
]. On peut identifier/modifier l'installation de Maven et/ou fixer le fichiersettings.xml
.
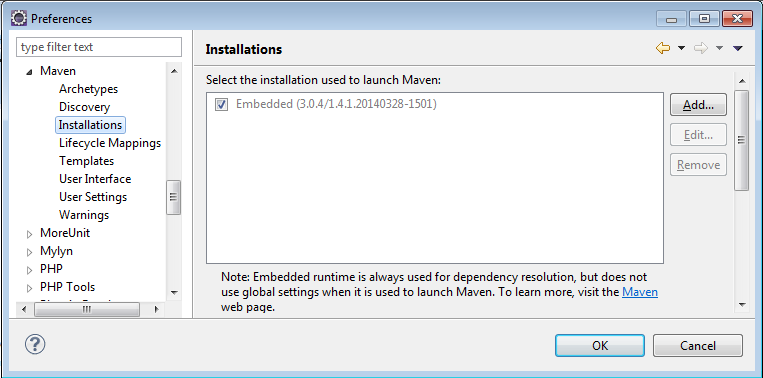
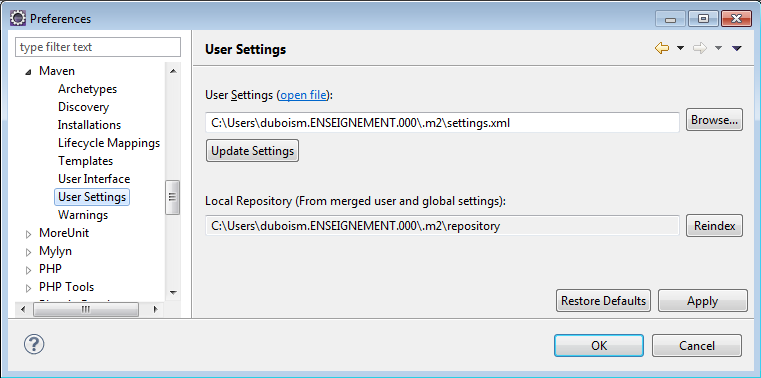
- Pour lancer, dans le menu
Run As
, une phase de maven. - Pour éditer un fichier
pom.xml
avec l’onglet du même nom de l'éditeur Pom. L’ongletDependency Hierarchy
remplace l’ancien ongletDependency Graph
. Il permet de voir l’arborescence des dépendances. Assurez-vous d'ouvrir le fichier pom.xml en utilisant l'éditeur de POM. Cliquez-droit sur le fichier, puis sélectionnezOpen with...
>Maven POM Editor
. Cela devrait vous donner l'éditeur attendu avec l'option pour afficher les dépendances et la hiérarchie de dépendance.
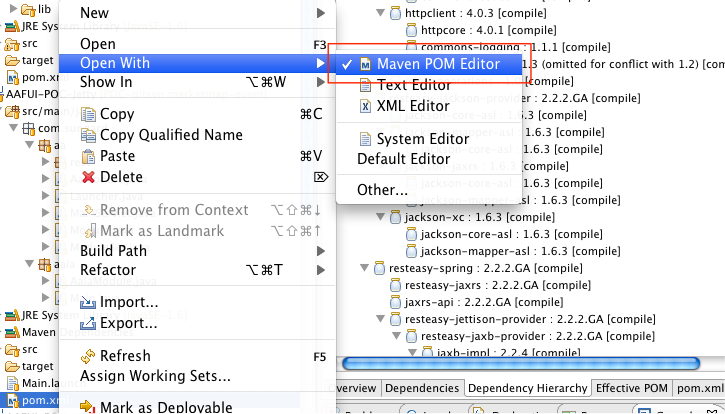
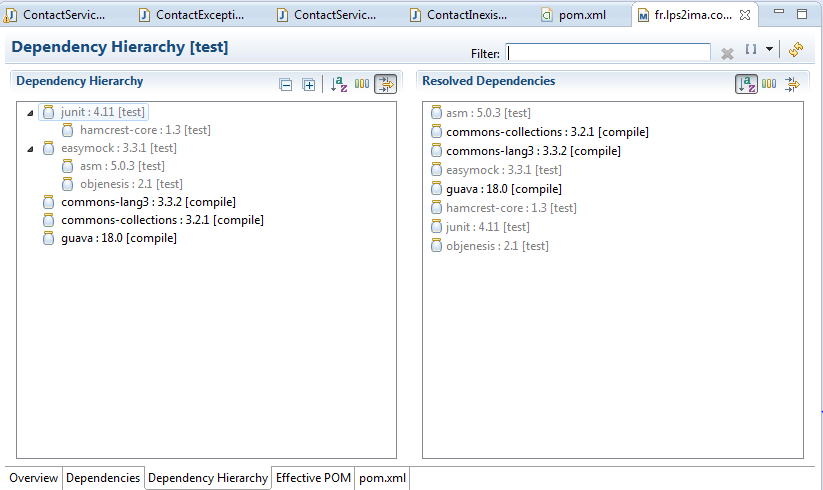
Recommandation : Utiliser pour un nouveau projet la commande File
> New
> Maven Project
.
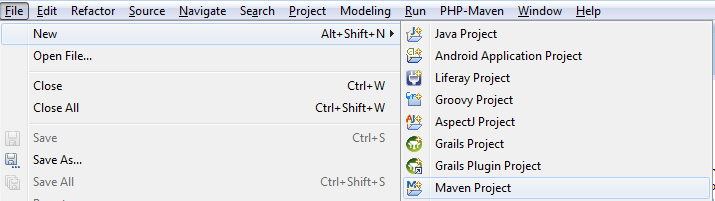
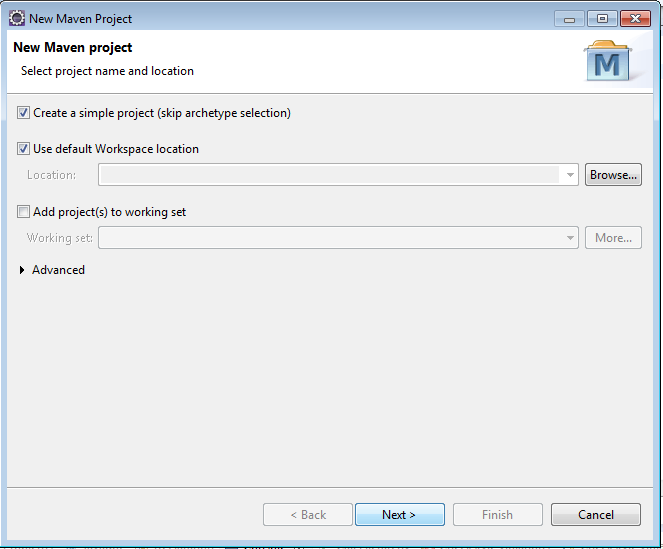
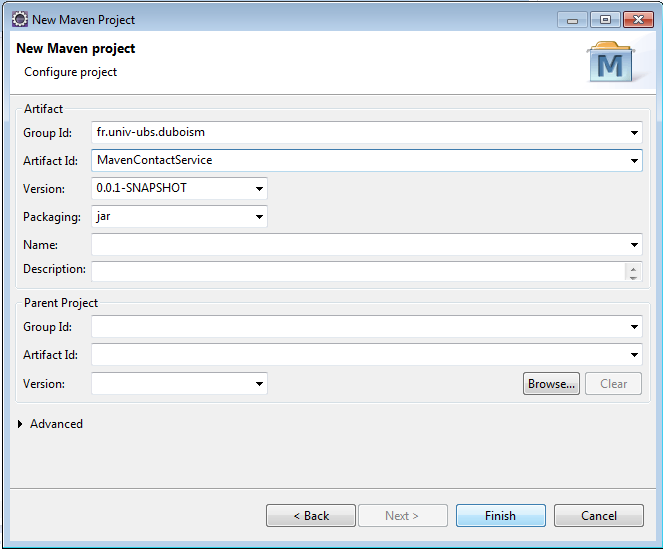
A la racine du nouveau projet un fichier pom.xml
a été généré.
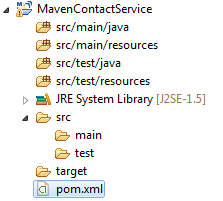
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>fr.univ-ubs.duboism</groupId> <artifactId>MavenContactService</artifactId> <version>0.0.1-SNAPSHOT</version> </project>
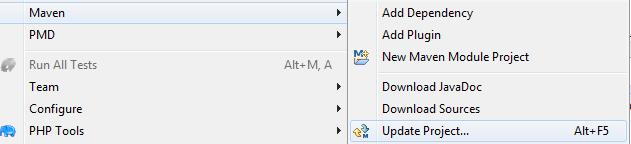
Avec l'éditeur de pom.xml
(sélectionner ce fichier, clic droit, Open With
> Maven POM Editor
), onglet Dependencies', on appuit sur le bouton Add
.
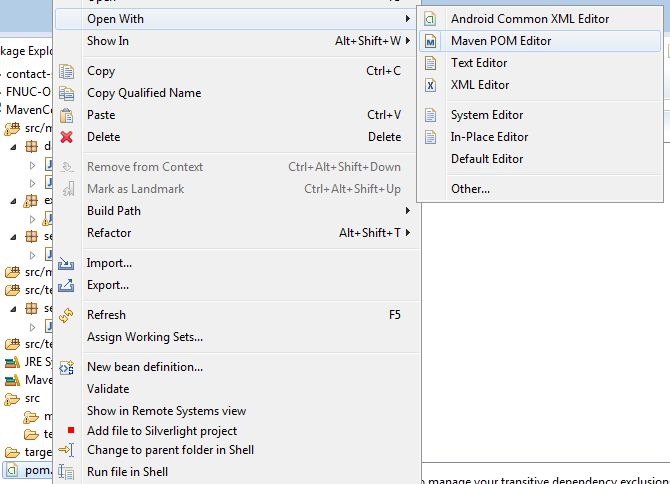
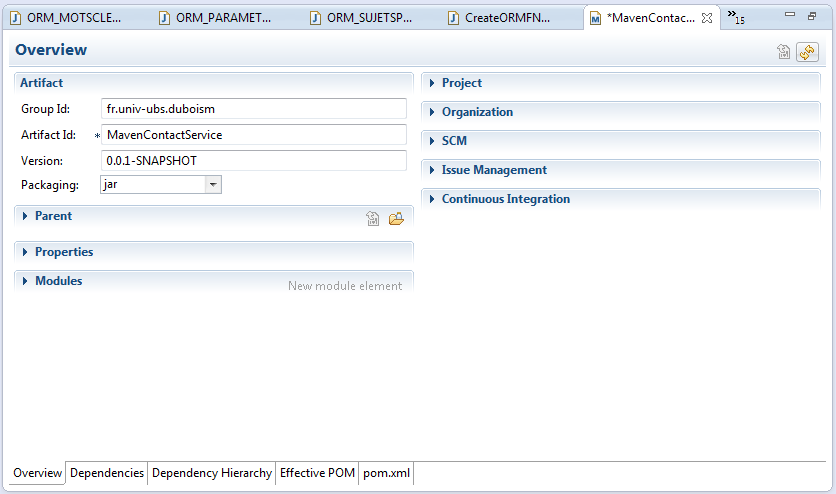
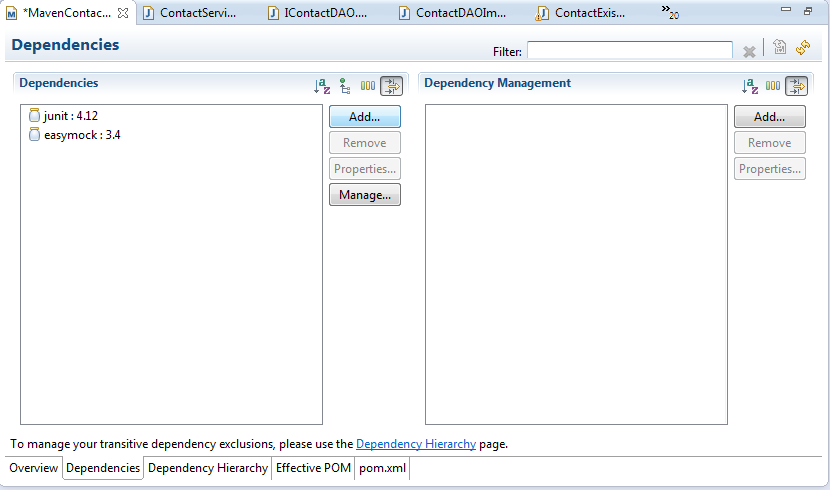
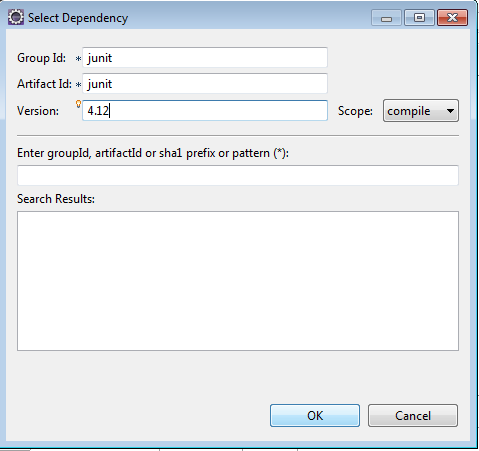
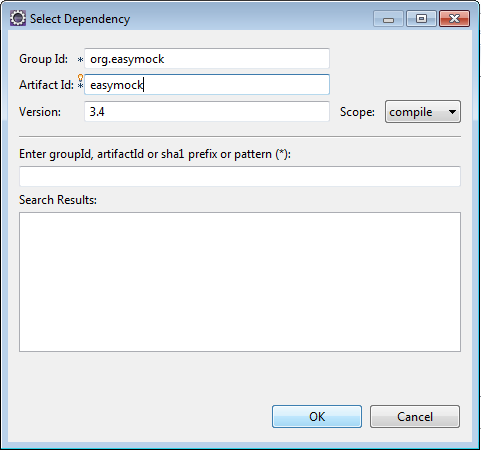
L'onglet pom.xml nous donne alors :
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>fr.univ-ubs.duboism</groupId> <artifactId>MavenContactService</artifactId> <version>0.0.1-SNAPSHOT</version> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <dependency> <groupId>org.easymock</groupId> <artifactId>easymock</artifactId> <version>3.4</version> </dependency> </dependencies> </project>
On peut aussi ajouter la dépendance guava :
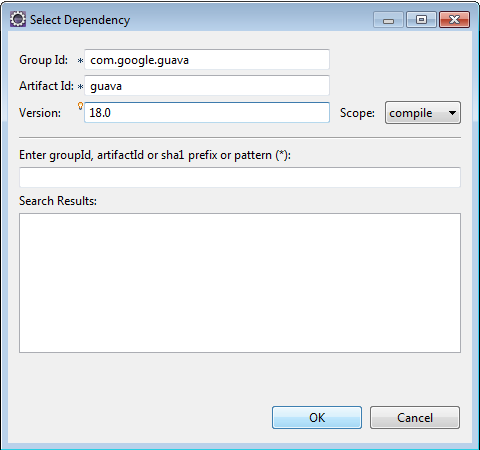
On modifie nos classes pour utiliser ces bibliothèques et on update le projet avec Maven.
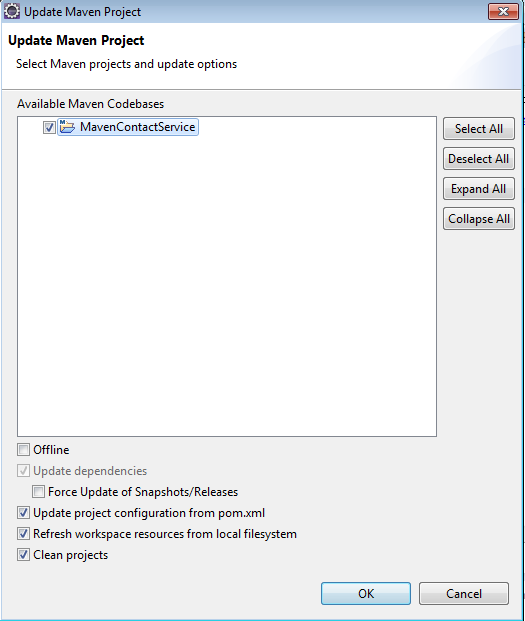
Les dépendances Maven sont ajoutées au projet :
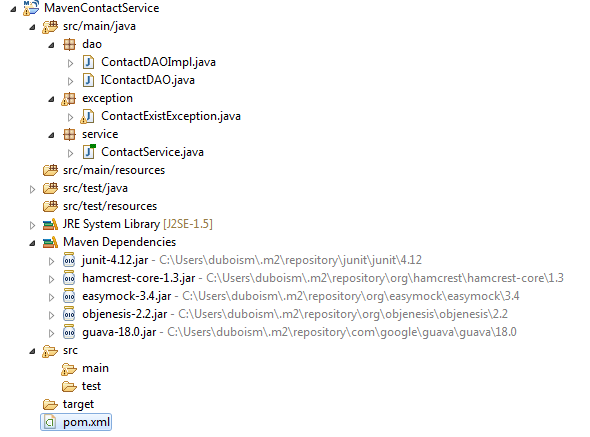
Première édition du fichier pom.xml
d'un projet Eclipse Java (qui n'est pas Maven à l'origine)
pom.xml
à la racine du projet Eclipse contact-service
dans le workspace.
<project> <modelVersion>4.0.0</modelVersion> <name>Contact</name> <artifactId>fr.lps2ima.contact</artifactId> <groupId>fr.lps2ima</groupId> <version>1.0.0-SNAPSHOT</version> <properties> <junit.version>4.11</junit.version> <easymock.version>3.3.1</easymock.version> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.easymock</groupId> <artifactId>easymock</artifactId> <version>${easymock.version}</version> <scope>test</scope> </dependency> </dependencies> </project>
Menu Run
/ Run Configurations
.
- Choisir
Maven Build
/New_configuration
. - Au niveau de
Name
:eclipse-eclipse
. - Au niveau de
Base directory
:${workspace_loc:/contact-service}
(FaireBrowse Workspace...
et sélectionner le projet pour obtenir cette valeur). - Au niveau de
Goals
:eclipse:clean eclipse:eclipse
. - Appuyer sur le bouton
Run
.
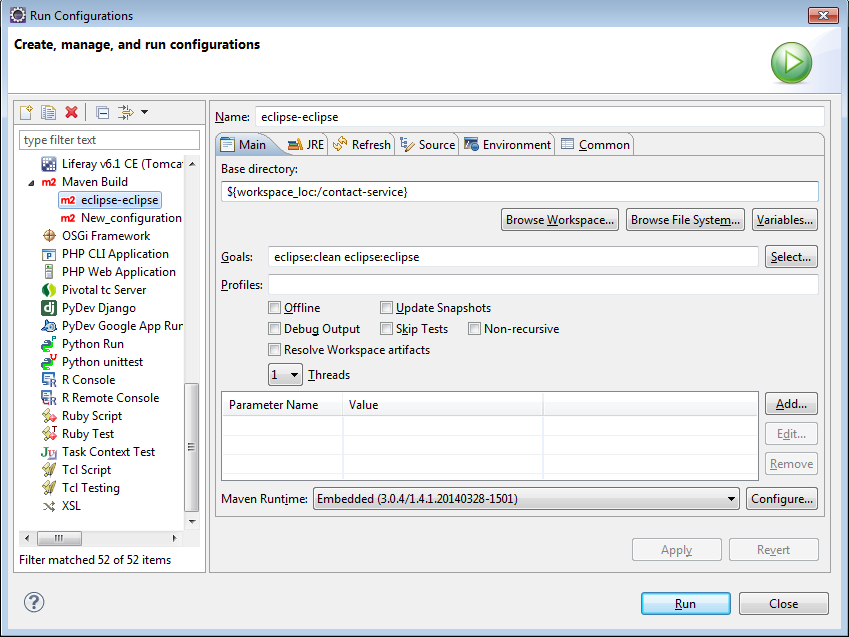
Menu Run
/ Run Configurations
.
- Choisir
Maven Build
/New_configuration
. - Au niveau de
Name
:maven-test
. - Au niveau de
Base directory
:${workspace_loc:/contact-service}
(FaireBrowse Workspace...
et sélectionner le projet pour obtenir cette valeur). - Au niveau de
Goals
:test
. - Appuyer sur le bouton
Run
.
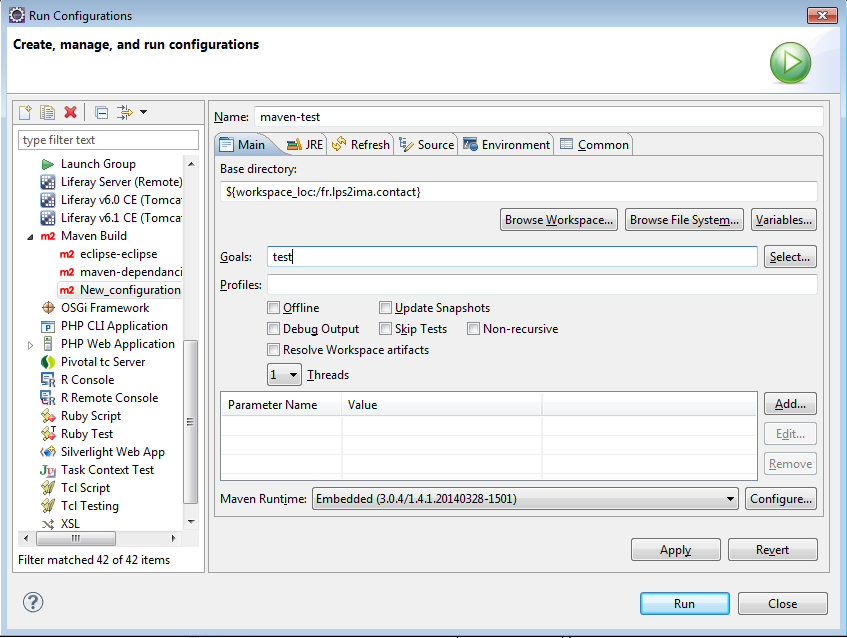
Après l'exécution, avec la commande Refresh
du projet, apparaissent des bibliothèques référencées.

SLF4J: Failed to load class "org.slf4j.impl.StaticLoggerBinder". SLF4J: Defaulting to no-operation (NOP) logger implementation SLF4J: See http://www.slf4j.org/codes.html#StaticLoggerBinder for further details. [INFO] Scanning for projects... Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-clean-plugin/2.4.1/maven-clean-plugin-2.4.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-clean-plugin/2.4.1/maven-clean-plugin-2.4.1.pom (5 KB at 0.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/18/maven-plugins-18.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/18/maven-plugins-18.pom (13 KB at 409.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/16/maven-parent-16.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/16/maven-parent-16.pom (23 KB at 483.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/apache/7/apache-7.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/apache/7/apache-7.pom (15 KB at 454.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-clean-plugin/2.4.1/maven-clean-plugin-2.4.1.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-clean-plugin/2.4.1/maven-clean-plugin-2.4.1.jar (23 KB at 489.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-install-plugin/2.3.1/maven-install-plugin-2.3.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-install-plugin/2.3.1/maven-install-plugin-2.3.1.pom (5 KB at 153.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-install-plugin/2.3.1/maven-install-plugin-2.3.1.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-install-plugin/2.3.1/maven-install-plugin-2.3.1.jar (23 KB at 717.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-resources-plugin/2.5/maven-resources-plugin-2.5.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-resources-plugin/2.5/maven-resources-plugin-2.5.pom (7 KB at 224.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/19/maven-plugins-19.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/19/maven-plugins-19.pom (11 KB at 335.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/19/maven-parent-19.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/19/maven-parent-19.pom (25 KB at 787.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/apache/9/apache-9.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/apache/9/apache-9.pom (15 KB at 477.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-resources-plugin/2.5/maven-resources-plugin-2.5.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-resources-plugin/2.5/maven-resources-plugin-2.5.jar (26 KB at 798.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-surefire-plugin/2.10/maven-surefire-plugin-2.10.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-surefire-plugin/2.10/maven-surefire-plugin-2.10.pom (11 KB at 339.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire/2.10/surefire-2.10.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire/2.10/surefire-2.10.pom (12 KB at 375.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/20/maven-parent-20.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/20/maven-parent-20.pom (25 KB at 775.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-surefire-plugin/2.10/maven-surefire-plugin-2.10.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-surefire-plugin/2.10/maven-surefire-plugin-2.10.jar (30 KB at 948.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-compiler-plugin/2.3.2/maven-compiler-plugin-2.3.2.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-compiler-plugin/2.3.2/maven-compiler-plugin-2.3.2.pom (8 KB at 230.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-compiler-plugin/2.3.2/maven-compiler-plugin-2.3.2.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-compiler-plugin/2.3.2/maven-compiler-plugin-2.3.2.jar (29 KB at 919.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-jar-plugin/2.3.2/maven-jar-plugin-2.3.2.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-jar-plugin/2.3.2/maven-jar-plugin-2.3.2.pom (6 KB at 90.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/21/maven-plugins-21.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/21/maven-plugins-21.pom (13 KB at 380.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-jar-plugin/2.3.2/maven-jar-plugin-2.3.2.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-jar-plugin/2.3.2/maven-jar-plugin-2.3.2.jar (32 KB at 1999.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-deploy-plugin/2.7/maven-deploy-plugin-2.7.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-deploy-plugin/2.7/maven-deploy-plugin-2.7.pom (6 KB at 176.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/22/maven-plugins-22.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/22/maven-plugins-22.pom (13 KB at 410.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/21/maven-parent-21.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/21/maven-parent-21.pom (26 KB at 830.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/apache/10/apache-10.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/apache/10/apache-10.pom (15 KB at 466.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-deploy-plugin/2.7/maven-deploy-plugin-2.7.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-deploy-plugin/2.7/maven-deploy-plugin-2.7.jar (27 KB at 569.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-site-plugin/3.0/maven-site-plugin-3.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-site-plugin/3.0/maven-site-plugin-3.0.pom (20 KB at 639.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-site-plugin/3.0/maven-site-plugin-3.0.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-site-plugin/3.0/maven-site-plugin-3.0.jar (112 KB at 1768.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-antrun-plugin/1.3/maven-antrun-plugin-1.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-antrun-plugin/1.3/maven-antrun-plugin-1.3.pom (5 KB at 149.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/12/maven-plugins-12.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/12/maven-plugins-12.pom (12 KB at 358.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/9/maven-parent-9.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/9/maven-parent-9.pom (33 KB at 1034.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/apache/4/apache-4.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/apache/4/apache-4.pom (5 KB at 141.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-antrun-plugin/1.3/maven-antrun-plugin-1.3.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-antrun-plugin/1.3/maven-antrun-plugin-1.3.jar (24 KB at 719.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-assembly-plugin/2.2-beta-5/maven-assembly-plugin-2.2-beta-5.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-assembly-plugin/2.2-beta-5/maven-assembly-plugin-2.2-beta-5.pom (15 KB at 462.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/16/maven-plugins-16.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-plugins/16/maven-plugins-16.pom (13 KB at 407.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/15/maven-parent-15.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/15/maven-parent-15.pom (24 KB at 1562.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/apache/6/apache-6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/apache/6/apache-6.pom (13 KB at 781.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-assembly-plugin/2.2-beta-5/maven-assembly-plugin-2.2-beta-5.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-assembly-plugin/2.2-beta-5/maven-assembly-plugin-2.2-beta-5.jar (204 KB at 2615.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-dependency-plugin/2.1/maven-dependency-plugin-2.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-dependency-plugin/2.1/maven-dependency-plugin-2.1.pom (8 KB at 256.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-dependency-plugin/2.1/maven-dependency-plugin-2.1.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-dependency-plugin/2.1/maven-dependency-plugin-2.1.jar (104 KB at 2252.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-release-plugin/2.0/maven-release-plugin-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-release-plugin/2.0/maven-release-plugin-2.0.pom (8 KB at 238.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/release/maven-release/2.0/maven-release-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/release/maven-release/2.0/maven-release-2.0.pom (7 KB at 216.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-release-plugin/2.0/maven-release-plugin-2.0.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-release-plugin/2.0/maven-release-plugin-2.0.jar (38 KB at 1213.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-metadata.xml Downloading: http://repo.maven.apache.org/maven2/org/codehaus/mojo/maven-metadata.xml Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-metadata.xml (13 KB at 407.2 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/mojo/maven-metadata.xml (20 KB at 314.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-eclipse-plugin/maven-metadata.xml Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-eclipse-plugin/maven-metadata.xml (709 B at 43.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-eclipse-plugin/2.9/maven-eclipse-plugin-2.9.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-eclipse-plugin/2.9/maven-eclipse-plugin-2.9.pom (15 KB at 475.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-eclipse-plugin/2.9/maven-eclipse-plugin-2.9.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-eclipse-plugin/2.9/maven-eclipse-plugin-2.9.jar (213 KB at 1362.6 KB/sec) [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building Contact 1.0.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-eclipse-plugin:2.9:clean (default-cli) @ fr.lps2im.contact --- Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-project/2.0.8/maven-project-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-project/2.0.8/maven-project-2.0.8.pom (3 KB at 165.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven/2.0.8/maven-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven/2.0.8/maven-2.0.8.pom (12 KB at 381.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/6/maven-parent-6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/6/maven-parent-6.pom (20 KB at 611.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-settings/2.0.8/maven-settings-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-settings/2.0.8/maven-settings-2.0.8.pom (3 KB at 125.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-model/2.0.8/maven-model-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-model/2.0.8/maven-model-2.0.8.pom (4 KB at 204.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.4.6/plexus-utils-1.4.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.4.6/plexus-utils-1.4.6.pom (3 KB at 48.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.11/plexus-1.0.11.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.11/plexus-1.0.11.pom (9 KB at 282.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-container-default/1.0-alpha-9-stable-1/plexus-container-default-1.0-alpha-9-stable-1.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-container-default/1.0-alpha-9-stable-1/plexus-container-default-1.0-alpha-9-stable-1.pom (4 KB at 124.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-containers/1.0.3/plexus-containers-1.0.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-containers/1.0.3/plexus-containers-1.0.3.pom (492 B at 15.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.4/plexus-1.0.4.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.4/plexus-1.0.4.pom (6 KB at 373.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/junit/junit/3.8.1/junit-3.8.1.pom Downloaded: http://repo.maven.apache.org/maven2/junit/junit/3.8.1/junit-3.8.1.pom (998 B at 21.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.0.4/plexus-utils-1.0.4.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.0.4/plexus-utils-1.0.4.pom (7 KB at 209.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/classworlds/classworlds/1.1-alpha-2/classworlds-1.1-alpha-2.pom Downloaded: http://repo.maven.apache.org/maven2/classworlds/classworlds/1.1-alpha-2/classworlds-1.1-alpha-2.pom (4 KB at 98.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-profile/2.0.8/maven-profile-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-profile/2.0.8/maven-profile-2.0.8.pom (2 KB at 64.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact-manager/2.0.8/maven-artifact-manager-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact-manager/2.0.8/maven-artifact-manager-2.0.8.pom (3 KB at 57.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-repository-metadata/2.0.8/maven-repository-metadata-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-repository-metadata/2.0.8/maven-repository-metadata-2.0.8.pom (2 KB at 59.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact/2.0.8/maven-artifact-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact/2.0.8/maven-artifact-2.0.8.pom (2 KB at 105.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-registry/2.0.8/maven-plugin-registry-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-registry/2.0.8/maven-plugin-registry-2.0.8.pom (2 KB at 122.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-api/2.0.8/maven-plugin-api-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-api/2.0.8/maven-plugin-api-2.0.8.pom (2 KB at 97.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-core/2.0.8/maven-core-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-core/2.0.8/maven-core-2.0.8.pom (7 KB at 212.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-parameter-documenter/2.0.8/maven-plugin-parameter-documenter-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-parameter-documenter/2.0.8/maven-plugin-parameter-documenter-2.0.8.pom (2 KB at 61.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/reporting/maven-reporting-api/2.0.8/maven-reporting-api-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/reporting/maven-reporting-api/2.0.8/maven-reporting-api-2.0.8.pom (2 KB at 56.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/reporting/maven-reporting/2.0.8/maven-reporting-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/reporting/maven-reporting/2.0.8/maven-reporting-2.0.8.pom (2 KB at 46.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/doxia/doxia-sink-api/1.0-alpha-9/doxia-sink-api-1.0-alpha-9.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/doxia/doxia-sink-api/1.0-alpha-9/doxia-sink-api-1.0-alpha-9.pom (2 KB at 82.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/doxia/doxia/1.0-alpha-9/doxia-1.0-alpha-9.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/doxia/doxia/1.0-alpha-9/doxia-1.0-alpha-9.pom (9 KB at 279.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-error-diagnostics/2.0.8/maven-error-diagnostics-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-error-diagnostics/2.0.8/maven-error-diagnostics-2.0.8.pom (2 KB at 53.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/commons-cli/commons-cli/1.0/commons-cli-1.0.pom Downloaded: http://repo.maven.apache.org/maven2/commons-cli/commons-cli/1.0/commons-cli-1.0.pom (3 KB at 64.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-descriptor/2.0.8/maven-plugin-descriptor-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-descriptor/2.0.8/maven-plugin-descriptor-2.0.8.pom (3 KB at 65.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-api/1.0-alpha-4/plexus-interactivity-api-1.0-alpha-4.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-api/1.0-alpha-4/plexus-interactivity-api-1.0-alpha-4.pom (7 KB at 223.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-monitor/2.0.8/maven-monitor-2.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-monitor/2.0.8/maven-monitor-2.0.8.pom (2 KB at 40.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/classworlds/classworlds/1.1/classworlds-1.1.pom Downloaded: http://repo.maven.apache.org/maven2/classworlds/classworlds/1.1/classworlds-1.1.pom (4 KB at 104.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/wagon/wagon-provider-api/2.1/wagon-provider-api-2.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/wagon/wagon-provider-api/2.1/wagon-provider-api-2.1.pom (2 KB at 36.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/wagon/wagon/2.1/wagon-2.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/wagon/wagon/2.1/wagon-2.1.pom (16 KB at 497.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/3.0/plexus-utils-3.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/3.0/plexus-utils-3.0.pom (4 KB at 128.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/sonatype/spice/spice-parent/16/spice-parent-16.pom Downloaded: http://repo.maven.apache.org/maven2/org/sonatype/spice/spice-parent/16/spice-parent-16.pom (9 KB at 263.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/sonatype/forge/forge-parent/5/forge-parent-5.pom Downloaded: http://repo.maven.apache.org/maven2/org/sonatype/forge/forge-parent/5/forge-parent-5.pom (9 KB at 255.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/commons-io/commons-io/1.4/commons-io-1.4.pom Downloaded: http://repo.maven.apache.org/maven2/commons-io/commons-io/1.4/commons-io-1.4.pom (13 KB at 414.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/commons/commons-parent/7/commons-parent-7.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/commons/commons-parent/7/commons-parent-7.pom (18 KB at 551.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-archiver/1.0-alpha-7/plexus-archiver-1.0-alpha-7.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-archiver/1.0-alpha-7/plexus-archiver-1.0-alpha-7.pom (2 KB at 32.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-components/1.1.6/plexus-components-1.1.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-components/1.1.6/plexus-components-1.1.6.pom (2 KB at 59.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.8/plexus-1.0.8.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.8/plexus-1.0.8.pom (8 KB at 227.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.2/plexus-utils-1.2.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.2/plexus-utils-1.2.pom (767 B at 24.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.5/plexus-1.0.5.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.5/plexus-1.0.5.pom (6 KB at 181.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-container-default/1.0-alpha-8/plexus-container-default-1.0-alpha-8.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-container-default/1.0-alpha-8/plexus-container-default-1.0-alpha-8.pom (8 KB at 228.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.5.6/plexus-utils-1.5.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.5.6/plexus-utils-1.5.6.pom (6 KB at 161.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.12/plexus-1.0.12.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/1.0.12/plexus-1.0.12.pom (10 KB at 308.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-jline/1.0-alpha-5/plexus-interactivity-jline-1.0-alpha-5.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-jline/1.0-alpha-5/plexus-interactivity-jline-1.0-alpha-5.pom (772 B at 24.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity/1.0-alpha-5/plexus-interactivity-1.0-alpha-5.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity/1.0-alpha-5/plexus-interactivity-1.0-alpha-5.pom (482 B at 15.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-components/1.1.4/plexus-components-1.1.4.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-components/1.1.4/plexus-components-1.1.4.pom (3 KB at 63.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/jline/jline/0.9.1/jline-0.9.1.pom Downloaded: http://repo.maven.apache.org/maven2/jline/jline/0.9.1/jline-0.9.1.pom (145 B at 4.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-api/1.0-alpha-5/plexus-interactivity-api-1.0-alpha-5.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-api/1.0-alpha-5/plexus-interactivity-api-1.0-alpha-5.pom (430 B at 13.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-archiver/2.2/maven-archiver-2.2.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-archiver/2.2/maven-archiver-2.2.pom (2 KB at 1268.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-shared-components/3/maven-shared-components-3.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-shared-components/3/maven-shared-components-3.pom (2 KB at 62.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/4/maven-parent-4.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/4/maven-parent-4.pom (10 KB at 305.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/apache/3/apache-3.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/apache/3/apache-3.pom (4 KB at 108.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-project/2.0/maven-project-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-project/2.0/maven-project-2.0.pom (2 KB at 51.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven/2.0/maven-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven/2.0/maven-2.0.pom (9 KB at 276.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-profile/2.0/maven-profile-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-profile/2.0/maven-profile-2.0.pom (2 KB at 83.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-model/2.0/maven-model-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-model/2.0/maven-model-2.0.pom (3 KB at 75.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact-manager/2.0/maven-artifact-manager-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact-manager/2.0/maven-artifact-manager-2.0.pom (2 KB at 42.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-repository-metadata/2.0/maven-repository-metadata-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-repository-metadata/2.0/maven-repository-metadata-2.0.pom (2 KB at 74.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact/2.0/maven-artifact-2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact/2.0/maven-artifact-2.0.pom (723 B at 47.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-resources/1.0-alpha-7/plexus-resources-1.0-alpha-7.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-resources/1.0-alpha-7/plexus-resources-1.0-alpha-7.pom (2 KB at 42.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-components/1.1.17/plexus-components-1.1.17.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-components/1.1.17/plexus-components-1.1.17.pom (6 KB at 164.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/2.0.5/plexus-2.0.5.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus/2.0.5/plexus-2.0.5.pom (17 KB at 1129.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.5.5/plexus-utils-1.5.5.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.5.5/plexus-utils-1.5.5.pom (6 KB at 162.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/biz/aQute/bndlib/0.0.145/bndlib-0.0.145.pom Downloaded: http://repo.maven.apache.org/maven2/biz/aQute/bndlib/0.0.145/bndlib-0.0.145.pom (886 B at 27.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-osgi/0.2.0/maven-osgi-0.2.0.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-osgi/0.2.0/maven-osgi-0.2.0.pom (2 KB at 64.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-shared-components/8/maven-shared-components-8.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-shared-components/8/maven-shared-components-8.pom (3 KB at 81.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/7/maven-parent-7.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/7/maven-parent-7.pom (21 KB at 669.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-project/2.0.6/maven-project-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-project/2.0.6/maven-project-2.0.6.pom (3 KB at 83.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven/2.0.6/maven-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven/2.0.6/maven-2.0.6.pom (9 KB at 285.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/5/maven-parent-5.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-parent/5/maven-parent-5.pom (15 KB at 930.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-settings/2.0.6/maven-settings-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-settings/2.0.6/maven-settings-2.0.6.pom (2 KB at 130.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-model/2.0.6/maven-model-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-model/2.0.6/maven-model-2.0.6.pom (3 KB at 96.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.4.1/plexus-utils-1.4.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.4.1/plexus-utils-1.4.1.pom (2 KB at 60.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-profile/2.0.6/maven-profile-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-profile/2.0.6/maven-profile-2.0.6.pom (2 KB at 42.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact-manager/2.0.6/maven-artifact-manager-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact-manager/2.0.6/maven-artifact-manager-2.0.6.pom (3 KB at 82.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-repository-metadata/2.0.6/maven-repository-metadata-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-repository-metadata/2.0.6/maven-repository-metadata-2.0.6.pom (2 KB at 58.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact/2.0.6/maven-artifact-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-artifact/2.0.6/maven-artifact-2.0.6.pom (2 KB at 49.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-registry/2.0.6/maven-plugin-registry-2.0.6.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-plugin-registry/2.0.6/maven-plugin-registry-2.0.6.pom (2 KB at 61.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/biz/aQute/bndlib/0.0.203/bndlib-0.0.203.pom Downloaded: http://repo.maven.apache.org/maven2/biz/aQute/bndlib/0.0.203/bndlib-0.0.203.pom (886 B at 27.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/eclipse/core/resources/3.3.0-v20070604/resources-3.3.0-v20070604.pom Downloaded: http://repo.maven.apache.org/maven2/org/eclipse/core/resources/3.3.0-v20070604/resources-3.3.0-v20070604.pom (2 KB at 35.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/junit/junit/3.8.1/junit-3.8.1.jar Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/reporting/maven-reporting-api/2.0.8/maven-reporting-api-2.0.8.jar Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/doxia/doxia-sink-api/1.0-alpha-9/doxia-sink-api-1.0-alpha-9.jar Downloading: http://repo.maven.apache.org/maven2/commons-cli/commons-cli/1.0/commons-cli-1.0.jar Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-api/1.0-alpha-4/plexus-interactivity-api-1.0-alpha-4.jar Downloading: http://repo.maven.apache.org/maven2/commons-io/commons-io/1.4/commons-io-1.4.jar Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-archiver/1.0-alpha-7/plexus-archiver-1.0-alpha-7.jar Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.5.6/plexus-utils-1.5.6.jar Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-jline/1.0-alpha-5/plexus-interactivity-jline-1.0-alpha-5.jar Downloading: http://repo.maven.apache.org/maven2/jline/jline/0.9.1/jline-0.9.1.jar Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/maven-archiver/2.2/maven-archiver-2.2.jar Downloading: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-resources/1.0-alpha-7/plexus-resources-1.0-alpha-7.jar Downloading: http://repo.maven.apache.org/maven2/biz/aQute/bndlib/0.0.145/bndlib-0.0.145.jar Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-osgi/0.2.0/maven-osgi-0.2.0.jar Downloading: http://repo.maven.apache.org/maven2/org/eclipse/core/resources/3.3.0-v20070604/resources-3.3.0-v20070604.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/reporting/maven-reporting-api/2.0.8/maven-reporting-api-2.0.8.jar (10 KB at 309.6 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/doxia/doxia-sink-api/1.0-alpha-9/doxia-sink-api-1.0-alpha-9.jar (10 KB at 153.6 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-api/1.0-alpha-4/plexus-interactivity-api-1.0-alpha-4.jar (14 KB at 207.8 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-interactivity-jline/1.0-alpha-5/plexus-interactivity-jline-1.0-alpha-5.jar (6 KB at 80.5 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/maven-archiver/2.2/maven-archiver-2.2.jar (10 KB at 155.3 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/shared/maven-osgi/0.2.0/maven-osgi-0.2.0.jar (13 KB at 156.8 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/junit/junit/3.8.1/junit-3.8.1.jar (119 KB at 1496.6 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/commons-cli/commons-cli/1.0/commons-cli-1.0.jar (30 KB at 377.1 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-resources/1.0-alpha-7/plexus-resources-1.0-alpha-7.jar (23 KB at 241.6 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/jline/jline/0.9.1/jline-0.9.1.jar (46 KB at 412.9 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/biz/aQute/bndlib/0.0.145/bndlib-0.0.145.jar (112 KB at 791.6 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-archiver/1.0-alpha-7/plexus-archiver-1.0-alpha-7.jar (139 KB at 889.2 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-utils/1.5.6/plexus-utils-1.5.6.jar (245 KB at 1308.4 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/commons-io/commons-io/1.4/commons-io-1.4.jar (107 KB at 195.0 KB/sec) Downloaded: http://repo.maven.apache.org/maven2/org/eclipse/core/resources/3.3.0-v20070604/resources-3.3.0-v20070604.jar (663 KB at 517.8 KB/sec) [INFO] Deleting file: .project [INFO] Deleting file: .classpath [INFO] Deleting file: .wtpmodules [INFO] [INFO] >>> maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2im.contact >>> [INFO] [INFO] <<< maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2im.contact <<< [INFO] [INFO] --- maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2im.contact --- [INFO] Using Eclipse Workspace: D:\workspace.4.2-64bits [WARNING] Workspace defines a VM that does not contain a valid jre/lib/rt.jar: D:\eclipse-jee-juno-SR2-win64\tools\liferay-portal-6.0.5\tomcat-6.0.26\jre1.6.0_21\win [INFO] no substring wtp server match. [INFO] Using as WTP server : Liferay v6.0 CE (Tomcat 6) [INFO] Adding default classpath container: org.eclipse.jdt.launching.JRE_CONTAINER Downloading: http://repo.maven.apache.org/maven2/junit/junit/4.11/junit-4.11.pom Downloaded: http://repo.maven.apache.org/maven2/junit/junit/4.11/junit-4.11.pom (3 KB at 73.8 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/hamcrest/hamcrest-core/1.3/hamcrest-core-1.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/hamcrest/hamcrest-core/1.3/hamcrest-core-1.3.pom (766 B at 24.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/hamcrest/hamcrest-parent/1.3/hamcrest-parent-1.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/hamcrest/hamcrest-parent/1.3/hamcrest-parent-1.3.pom (2 KB at 128.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/easymock/easymock/3.3.1/easymock-3.3.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/easymock/easymock/3.3.1/easymock-3.3.1.pom (5 KB at 129.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/easymock/easymock-parent/3.3.1/easymock-parent-3.3.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/easymock/easymock-parent/3.3.1/easymock-parent-3.3.1.pom (18 KB at 536.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/cglib/cglib/3.1/cglib-3.1.pom Downloaded: http://repo.maven.apache.org/maven2/cglib/cglib/3.1/cglib-3.1.pom (2 KB at 45.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/ow2/asm/asm/4.2/asm-4.2.pom Downloaded: http://repo.maven.apache.org/maven2/org/ow2/asm/asm/4.2/asm-4.2.pom (2 KB at 59.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/ow2/asm/asm-parent/4.2/asm-parent-4.2.pom Downloaded: http://repo.maven.apache.org/maven2/org/ow2/asm/asm-parent/4.2/asm-parent-4.2.pom (6 KB at 173.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/ow2/ow2/1.3/ow2-1.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/ow2/ow2/1.3/ow2-1.3.pom (10 KB at 619.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/ow2/asm/asm/5.0.3/asm-5.0.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/ow2/asm/asm/5.0.3/asm-5.0.3.pom (2 KB at 61.0 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/ow2/asm/asm-parent/5.0.3/asm-parent-5.0.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/ow2/asm/asm-parent/5.0.3/asm-parent-5.0.3.pom (6 KB at 173.1 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/objenesis/objenesis/2.1/objenesis-2.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/objenesis/objenesis/2.1/objenesis-2.1.pom (3 KB at 79.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/objenesis/objenesis-parent/2.1/objenesis-parent-2.1.pom Downloaded: http://repo.maven.apache.org/maven2/org/objenesis/objenesis-parent/2.1/objenesis-parent-2.1.pom (17 KB at 526.3 KB/sec) Downloading: http://repo.maven.apache.org/maven2/junit/junit/4.11/junit-4.11.jar Downloaded: http://repo.maven.apache.org/maven2/junit/junit/4.11/junit-4.11.jar (240 KB at 326.5 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/hamcrest/hamcrest-core/1.3/hamcrest-core-1.3.jar Downloaded: http://repo.maven.apache.org/maven2/org/hamcrest/hamcrest-core/1.3/hamcrest-core-1.3.jar (44 KB at 709.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/easymock/easymock/3.3.1/easymock-3.3.1.jar Downloaded: http://repo.maven.apache.org/maven2/org/easymock/easymock/3.3.1/easymock-3.3.1.jar (124 KB at 1585.6 KB/sec) Downloading: http://repo.maven.apache.org/maven2/cglib/cglib/3.1/cglib-3.1.jar Downloaded: http://repo.maven.apache.org/maven2/cglib/cglib/3.1/cglib-3.1.jar (277 KB at 2536.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/ow2/asm/asm/5.0.3/asm-5.0.3.jar Downloaded: http://repo.maven.apache.org/maven2/org/ow2/asm/asm/5.0.3/asm-5.0.3.jar (52 KB at 1676.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/objenesis/objenesis/2.1/objenesis-2.1.jar Downloaded: http://repo.maven.apache.org/maven2/org/objenesis/objenesis/2.1/objenesis-2.1.jar (41 KB at 1315.4 KB/sec) [INFO] Not writing settings - defaults suffice [INFO] Wrote Eclipse project for "fr.lps2im.contact" to D:\workspace.4.2-64bits\contact-service. [INFO] [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 18.437s [INFO] Finished at: Wed Jan 07 09:56:23 CET 2015 [INFO] Final Memory: 7M/97M [INFO] ------------------------------------------------------------------------
dependance la plus haute. dépendance transitve on peut exclure
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.3.2</version> </dependency>
SLF4J: Failed to load class "org.slf4j.impl.StaticLoggerBinder". SLF4J: Defaulting to no-operation (NOP) logger implementation SLF4J: See http://www.slf4j.org/codes.html#StaticLoggerBinder for further details. [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building Contact 1.0.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-eclipse-plugin:2.9:clean (default-cli) @ fr.lps2ima.contact --- [INFO] Deleting file: .project [INFO] Deleting file: .classpath [INFO] Deleting file: .wtpmodules [INFO] [INFO] >>> maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2ima.contact >>> [INFO] [INFO] <<< maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2ima.contact <<< [INFO] [INFO] --- maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2ima.contact --- [INFO] Using Eclipse Workspace: D:\workspace.4.2-64bits [WARNING] Workspace defines a VM that does not contain a valid jre/lib/rt.jar: D:\eclipse-jee-juno-SR2-win64\tools\liferay-portal-6.0.5\tomcat-6.0.26\jre1.6.0_21\win [INFO] no substring wtp server match. [INFO] Using as WTP server : Liferay v6.0 CE (Tomcat 6) [INFO] Adding default classpath container: org.eclipse.jdt.launching.JRE_CONTAINER Downloading: http://repo.maven.apache.org/maven2/org/apache/commons/commons-lang3/3.3.2/commons-lang3-3.3.2.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/commons/commons-lang3/3.3.2/commons-lang3-3.3.2.pom (20 KB at 3.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/commons/commons-parent/33/commons-parent-33.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/commons/commons-parent/33/commons-parent-33.pom (52 KB at 836.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/apache/13/apache-13.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/apache/13/apache-13.pom (14 KB at 440.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/commons/commons-lang3/3.3.2/commons-lang3-3.3.2.jar Downloaded: http://repo.maven.apache.org/maven2/org/apache/commons/commons-lang3/3.3.2/commons-lang3-3.3.2.jar (404 KB at 2858.6 KB/sec) [INFO] Not writing settings - defaults suffice [INFO] Wrote Eclipse project for "fr.lps2ima.contact" to D:\workspace.4.2-64bits\contact-service. [INFO] [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.549s [INFO] Finished at: Wed Jan 07 10:04:55 CET 2015 [INFO] Final Memory: 8M/78M [INFO] ------------------------------------------------------------------------
<dependency> <groupId>commons-collections</groupId> <artifactId>commons-collections</artifactId> <version>3.2.1</version> </dependency>
SLF4J: Failed to load class "org.slf4j.impl.StaticLoggerBinder". SLF4J: Defaulting to no-operation (NOP) logger implementation SLF4J: See http://www.slf4j.org/codes.html#StaticLoggerBinder for further details. [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building Contact 1.0.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-eclipse-plugin:2.9:clean (default-cli) @ fr.lps2ima.contact --- [INFO] Deleting file: .project [INFO] Deleting file: .classpath [INFO] Deleting file: .wtpmodules [INFO] [INFO] >>> maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2ima.contact >>> [INFO] [INFO] <<< maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2ima.contact <<< [INFO] [INFO] --- maven-eclipse-plugin:2.9:eclipse (default-cli) @ fr.lps2ima.contact --- [INFO] Using Eclipse Workspace: D:\workspace.4.2-64bits [WARNING] Workspace defines a VM that does not contain a valid jre/lib/rt.jar: D:\eclipse-jee-juno-SR2-win64\tools\liferay-portal-6.0.5\tomcat-6.0.26\jre1.6.0_21\win [INFO] no substring wtp server match. [INFO] Using as WTP server : Liferay v6.0 CE (Tomcat 6) [INFO] Adding default classpath container: org.eclipse.jdt.launching.JRE_CONTAINER Downloading: http://repo.maven.apache.org/maven2/commons-collections/commons-collections/3.2.1/commons-collections-3.2.1.pom Downloaded: http://repo.maven.apache.org/maven2/commons-collections/commons-collections/3.2.1/commons-collections-3.2.1.pom (13 KB at 2.4 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/apache/commons/commons-parent/9/commons-parent-9.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/commons/commons-parent/9/commons-parent-9.pom (22 KB at 356.9 KB/sec) Downloading: http://repo.maven.apache.org/maven2/commons-collections/commons-collections/3.2.1/commons-collections-3.2.1.jar Downloaded: http://repo.maven.apache.org/maven2/commons-collections/commons-collections/3.2.1/commons-collections-3.2.1.jar (562 KB at 2809.5 KB/sec) [INFO] Not writing settings - defaults suffice [INFO] Wrote Eclipse project for "fr.lps2ima.contact" to D:\workspace.4.2-64bits\contact-service. [INFO] [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 8.651s [INFO] Finished at: Wed Jan 07 10:08:21 CET 2015 [INFO] Final Memory: 12M/111M [INFO] ------------------------------------------------------------------------
Après l'exécution, avec la commande Refresh
du projet, apparaissent des bibliothèques référencées.

if(nom == null || nom.trim().length() < 3 || nom.trim().length()>40){
int taille=StringUtils.trimToEmpty(nom).length(); if (taille < 3 || taille>40){
Construire l'arbre de dépendances du fichier pom.xml
d'un projet Eclipse Java
Menu Run
/ Run Configurations
.
- Choisir
Maven Build
/New_configuration
. - Au niveau de
Name
:maven-dependancies
. - Au niveau de
Base directory
:${workspace_loc:/contact-service}
(FaireBrowse Workspace...
et sélectionner le projet pour obtenir cette valeur). - Au niveau de
Goals
:dependency:tree
. - Appuyer sur le bouton
Run
.
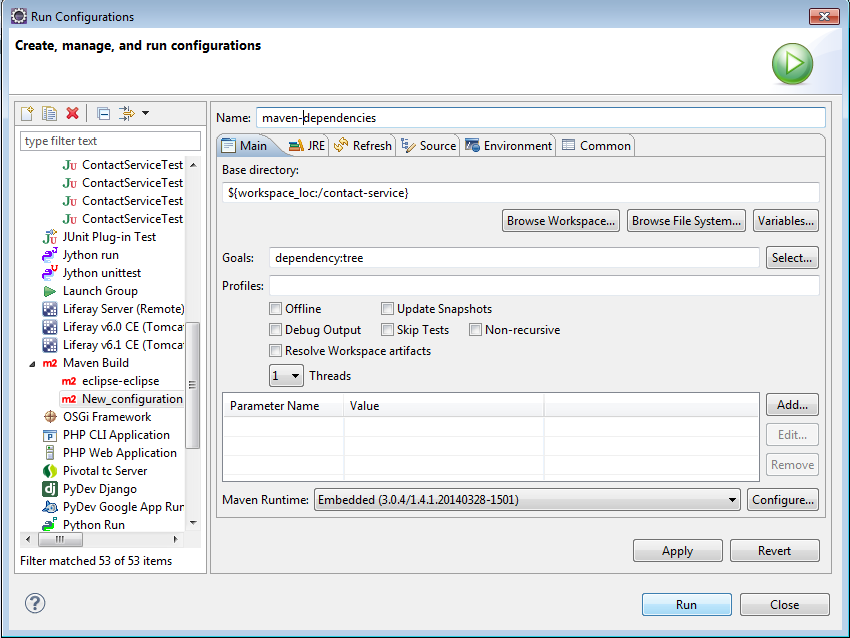
[INFO] fr.lps2ima:fr.lps2ima.contact:jar:1.0.0-SNAPSHOT [INFO] +- junit:junit:jar:4.11:test [INFO] | \- org.hamcrest:hamcrest-core:jar:1.3:test [INFO] +- org.easymock:easymock:jar:3.3.1:test [INFO] | +- cglib:cglib:jar:3.1:test [INFO] | +- org.ow2.asm:asm:jar:5.0.3:test [INFO] | \- org.objenesis:objenesis:jar:2.1:test [INFO] +- org.apache.commons:commons-lang3:jar:3.3.2:compile [INFO] \- commons-collections:commons-collections:jar:3.2.1:compile [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 14.372s [INFO] Finished at: Wed Jan 07 10:21:24 CET 2015 [INFO] Final Memory: 10M/288M [INFO] ------------------------------------------------------------------------
<dependency> <groupId>org.easymock</groupId> <artifactId>easymock</artifactId> <exclusions> <exclusion> <groupId>cglib</groupId> <artifactId>cglib</artifactId> </exclusion> </exclusions> <version>${easymock.version}</version> <scope>test</scope> </dependency>

cglib a disparu.
------------------------------------------------------- T E S T S ------------------------------------------------------- Running service.ContactServiceTest Tests run: 9, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 5.121 sec Results : Tests run: 9, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] --- maven-jar-plugin:2.3.2:jar (default-jar) @ fr.lps2ima.contact --- [INFO] [INFO] --- maven-install-plugin:2.3.1:install (default-install) @ fr.lps2ima.contact --- [INFO] Installing D:\workspace.4.2-64bits\contact-service\target\fr.lps2ima.contact-1.0.0-SNAPSHOT.jar to C:\Users\duboism\.m2\repository\fr\lps2ima\fr.lps2ima.contact\1.0.0-SNAPSHOT\fr.lps2ima.contact-1.0.0-SNAPSHOT.jar [INFO] Installing D:\workspace.4.2-64bits\contact-service\pom.xml to C:\Users\duboism\.m2\repository\fr\lps2ima\fr.lps2ima.contact\1.0.0-SNAPSHOT\fr.lps2ima.contact-1.0.0-SNAPSHOT.pom [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 11.764s [INFO] Finished at: Wed Jan 07 10:42:39 CET 2015 [INFO] Final Memory: 10M/107M [INFO] ------------------------------------------------------------------------
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-deploy-plugin:2.7:deploy (default-deploy) on project fr.lps2ima.contact: Deployment failed: repository element was not specified in the POM inside distributionManagement element or in -DaltDeploymentRepository=id::layout::url parameter -> [Help 1] [ERROR] [ERROR] To see the full stack trace of the errors, re-run Maven with the -e switch. [ERROR] Re-run Maven using the -X switch to enable full debug logging. [ERROR] [ERROR] For more information about the errors and possible solutions, please read the following articles: [ERROR] [Help 1] http://cwiki.apache.org/confluence/display/MAVEN/MojoExecutionException
<build> <plugins> <plugin> <inherited>true</inherited> <artifactId>maven-source-plugin</artifactId> <executions> <execution> <id>attach-sources</id> <goals> <goal>jar</goal> </goals> </execution> </executions> </plugin> <plugin> <inherited>true</inherited> <artifactId>maven-javadoc-plugin</artifactId> <executions> <execution> <id>attach-javadocs</id> <goals> <goal>jar</goal> </goals> </execution> </executions> </plugin> </plugins> </build>
[INFO] [INFO] --- maven-source-plugin:2.4:jar (attach-sources) @ fr.lps2ima.contact --- [INFO] [INFO] --- maven-javadoc-plugin:2.10.1:jar (attach-javadocs) @ fr.lps2ima.contact --- [WARNING] Source files encoding has not been set, using platform encoding Cp1252, i.e. build is platform dependent! [INFO] Loading source files for package dao... Loading source files for package exceptions... Loading source files for package model... Loading source files for package service... Constructing Javadoc information... Standard Doclet version 1.6.0_43 Building tree for all the packages and classes... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\ContactDaoImpl.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\IContactDao.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\exceptions/\ContactException.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\model/\Contact.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\service/\ContactService.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\overview-frame.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\package-frame.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\package-summary.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\package-tree.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\exceptions/\package-frame.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\exceptions/\package-summary.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\exceptions/\package-tree.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\model/\package-frame.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\model/\package-summary.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\model/\package-tree.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\service/\package-frame.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\service/\package-summary.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\service/\package-tree.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\constant-values.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\serialized-form.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\class-use\IContactDao.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\class-use\ContactDaoImpl.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\exceptions/\class-use\ContactException.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\model/\class-use\Contact.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\service/\class-use\ContactService.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\dao/\package-use.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\exceptions/\package-use.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\model/\package-use.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\service/\package-use.html... Building index for all the packages and classes... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\overview-tree.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\index-all.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\deprecated-list.html... Building index for all classes... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\allclasses-frame.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\allclasses-noframe.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\index.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\overview-summary.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\help-doc.html... Generating D:/workspace.4.2-64bits/contact-service/target/apidocs\stylesheet.css... 2 warnings [WARNING] Javadoc Warnings [WARNING] D:\workspace.4.2-64bits\contact-service\src\main\java\service\ContactService.java:8: package exception does not exist [WARNING] import exception.ContactInexistantException; [WARNING] ^ [WARNING] D:\workspace.4.2-64bits\contact-service\src\main\java\service\ContactService.java:37: cannot find symbol [WARNING] symbol : class ContactInexistantException [WARNING] location: class service.ContactService [WARNING] public Contact modifierNomContact(String ancienNom, String nouveauNom) throws ContactInexistantException, ContactException{ [WARNING] ^ [INFO] Fixed Javadoc frame injection vulnerability (CVE-2013-1571) in 1 files. [INFO] Building jar: D:\workspace.4.2-64bits\contact-service\target\fr.lps2ima.contact-1.0.0-SNAPSHOT-javadoc.jar [INFO] [INFO] --- maven-install-plugin:2.3.1:install (default-install) @ fr.lps2ima.contact --- [INFO] Installing D:\workspace.4.2-64bits\contact-service\target\fr.lps2ima.contact-1.0.0-SNAPSHOT.jar to C:\Users\duboism\.m2\repository\fr\lps2ima\fr.lps2ima.contact\1.0.0-SNAPSHOT\fr.lps2ima.contact-1.0.0-SNAPSHOT.jar [INFO] Installing D:\workspace.4.2-64bits\contact-service\pom.xml to C:\Users\duboism\.m2\repository\fr\lps2ima\fr.lps2ima.contact\1.0.0-SNAPSHOT\fr.lps2ima.contact-1.0.0-SNAPSHOT.pom [INFO] Installing D:\workspace.4.2-64bits\contact-service\target\fr.lps2ima.contact-1.0.0-SNAPSHOT-sources.jar to C:\Users\duboism\.m2\repository\fr\lps2ima\fr.lps2ima.contact\1.0.0-SNAPSHOT\fr.lps2ima.contact-1.0.0-SNAPSHOT-sources.jar [INFO] Installing D:\workspace.4.2-64bits\contact-service\target\fr.lps2ima.contact-1.0.0-SNAPSHOT-javadoc.jar to C:\Users\duboism\.m2\repository\fr\lps2ima\fr.lps2ima.contact\1.0.0-SNAPSHOT\fr.lps2ima.contact-1.0.0-SNAPSHOT-javadoc.jar [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 55.572s [INFO] Finished at: Wed Jan 07 11:03:00 CET 2015 [INFO] Final Memory: 11M/70M [INFO] ------------------------------------------------------------------------
[INFO] Scanning for projects... [WARNING] [WARNING] Some problems were encountered while building the effective model for fr.lps2ima:fr.lps2ima.contact:jar:1.0.0-SNAPSHOT [WARNING] 'build.plugins.plugin.version' for org.apache.maven.plugins:maven-javadoc-plugin is missing. @ line 63, column 19 [WARNING] 'build.plugins.plugin.version' for org.apache.maven.plugins:maven-source-plugin is missing. @ line 50, column 19 [WARNING] [WARNING] It is highly recommended to fix these problems because they threaten the stability of your build. [WARNING] [WARNING] For this reason, future Maven versions might no longer support building such malformed projects. [WARNING]
<plugin> <inherited>true</inherited> <artifactId>maven-source-plugin</artifactId> <version>2.4</version> <executions> <execution> <id>attach-sources</id> <goals> <goal>jar</goal> </goals> </execution> </executions> </plugin>
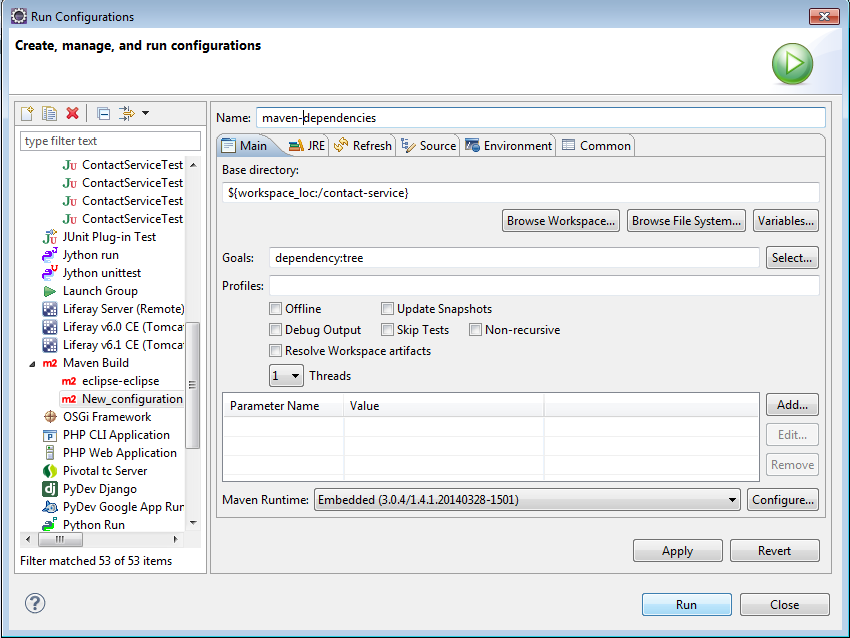

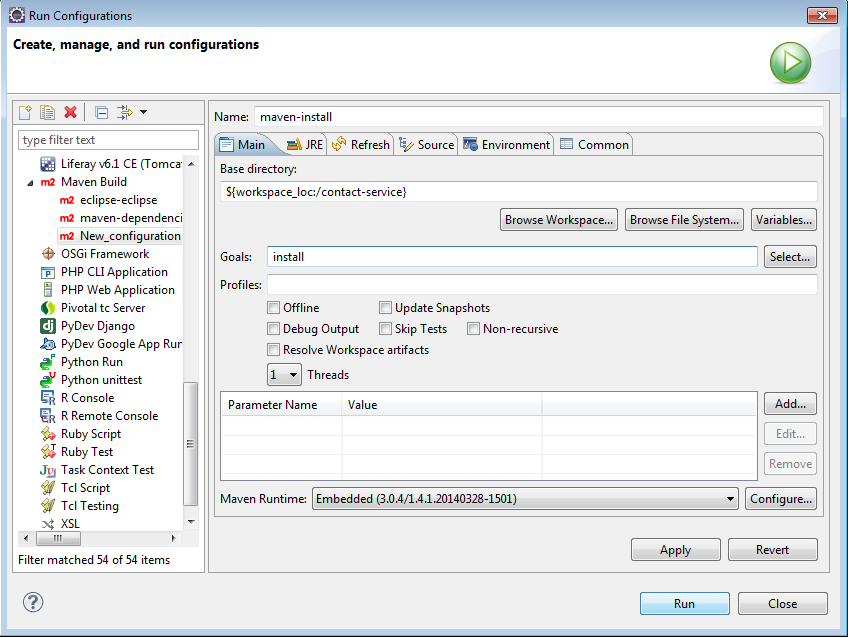
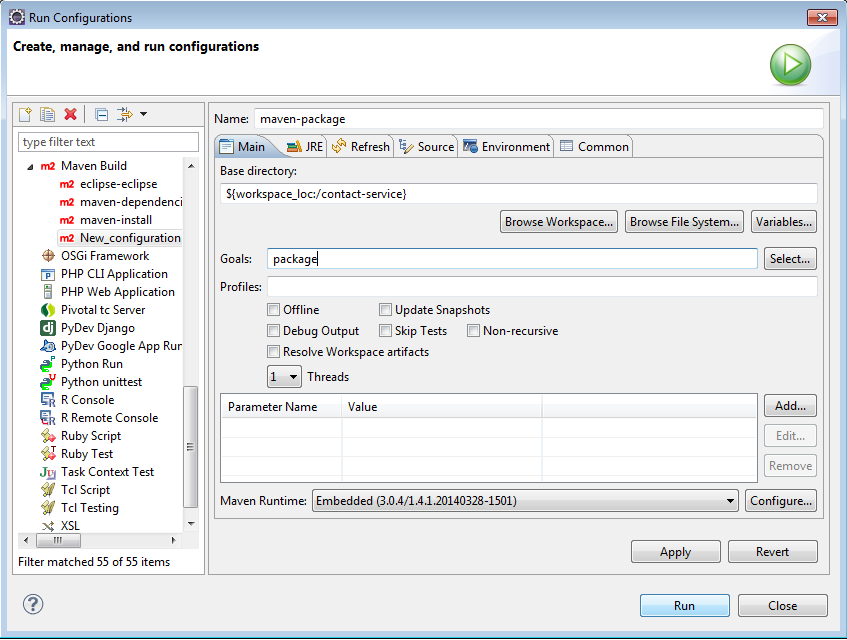
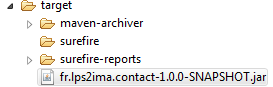
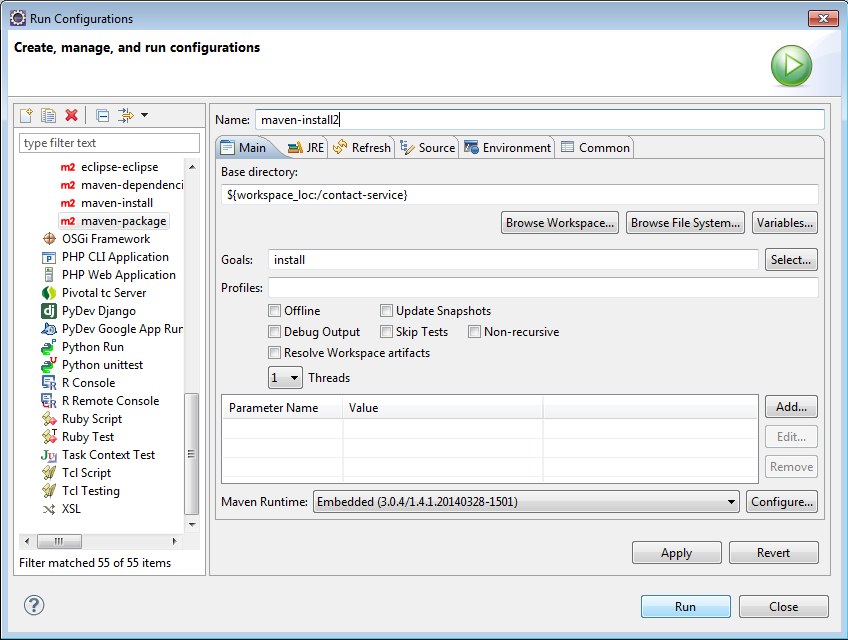
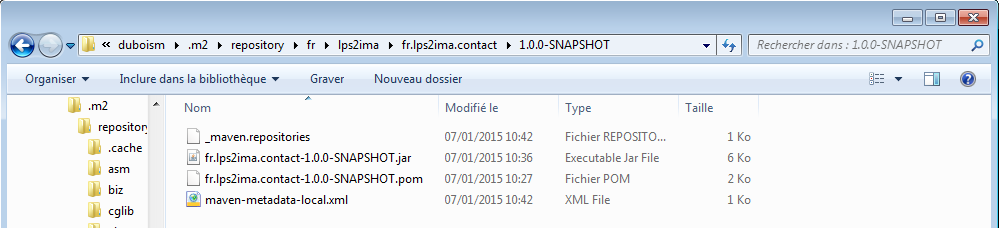
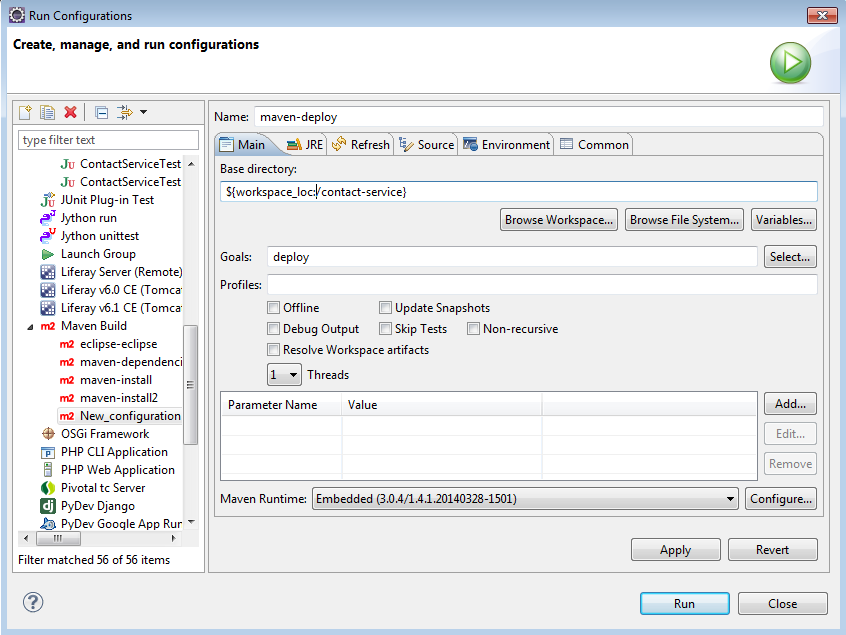
http://blog.netapsys.fr/m2eclipse-declarez-vos-projets-maven-dans-eclipse/
http://www.sitepoint.com/maven-and-php/
"Updating Maven Project". Unsupported IClasspathEntry kind=4
1 Right-click on your project, select Maven -> Remove Maven Nature.
2 Open you terminal, go to your project folder and do mvn eclipse:clean
3 Right click on your Project and select “Configure -> Convert into Maven Project”
Now you got “Unsupported IClasspathEntry kind=4 Eclipse Scala” disappear. shareimprove this answer
4
Thanks for this. Just a note for others, it's mvn eclipse:clean, not mvn clean (I got fooled by not paying close attention).
Have you tried:
If you have import project into the eclipse 4, please delete it. In maven consol, run: mvn eclipse:clean In Eclipse 4: File -> Import -> Maven -> Existing Maven Projects
before doing this, make sure maven eclipse plugin has not been run on the project previously. mvn eclipse:clean will fix it if needed. – AChoice Oct 11 '12 at 15:13 2
This worked better for me in Juno. I deleted the projects in Eclipse, and then re-imported. –
Comments
You actually expressed this wonderfully.
Best Essay writing
essay 5
You actually expressed this wonderfully.
Best Essay writing
essay 5
Regards! I value it.
Best Essay writing
essay writers
Regards! I value it.
Best Esssay writing
essaywriters
Amazing all kinds of ggreat advice!
Beest Essay writing
help essay writing
Amazing all kinds of great advice!
Best Essay writing
help essay writing
Greazt information Many thanks!
Best Essay writing
essay writers
Great information Manny thanks!
Best Essay writing
essay writers
Nicely voiced really! .
Best Essay writing
write papers for me
Nicely voiced really! .
Best Essay writing
write papers for me
Fantastic knowledge Thanks.
Best Essay writing
paper writing
Fantastic knowledge Thanks.
Best Essay writing
paper writing
You actually suggested that wonderfully!
Beest Esssy writing
buy essay help
Yoou actually suggested that wonderfully!
Best Essay writing
buy essay help
Seriously many of valuable data!
Best Essay writing
college essqy writing service
Seriously many of valuable data!
Bestt Essay writing
college essay writing service
You mentioned it exceptionally well.
Best Essay writing
write papers for me
You mentioned it exceptionally well.
Best Essay writing
write papers for me
Nicely put. Thanks a lot.
Best Essay writing
write mmy essay
Nicely put. Thanks a lot.
Best Essay writing
write my essay
Cheers. I appreciate it!
Best Essay writing
buy essay online for cheap
Cheers. I appreciate it!
Best Essay writing
buy essay online for cheap